The Unity SDK offers a streamlined bridge integration for the Android SDK, enhancing the ease of incorporating in-game purchase functionality within Unity projects.
This package simplifies the process of integrating the Android SDK file, providing a user-friendly interface and essential tools for seamless connectivity. With this Unity Package, developers can effortlessly enable native checkout experiences, ensuring smooth and secure transactions directly in-game.
Compatible with Unity’s development environment, it facilitates a quick setup, saving time and effort while supporting a range of transaction types. Enhance your game’s monetization and user experience with this efficient integration solution.
For an enhanced, immersive checkout experience in your mobile SDK, refer to the the Native Display Approach documentation once you’ve completed integrating the Unity SDK package.
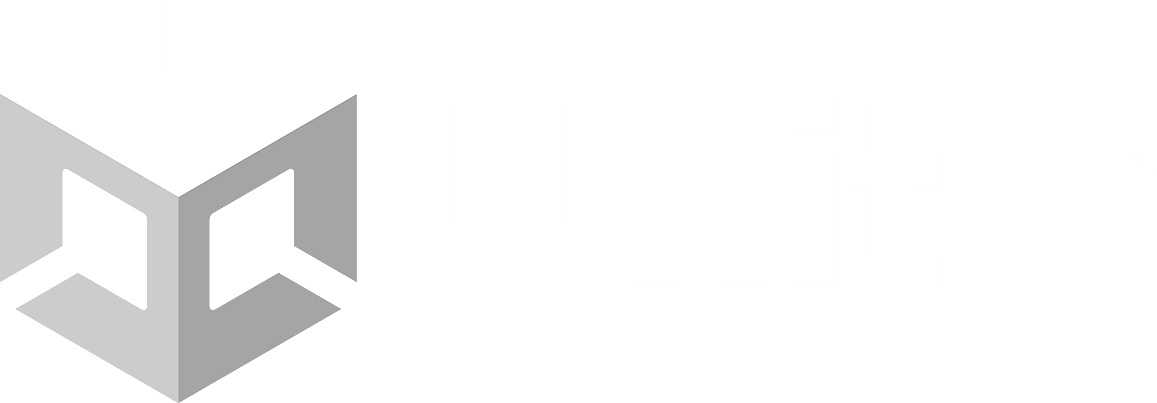