Overview
This document provides step-by-step instructions to help you integrate the SDK into your project. For further assistance, you can refer to the CheckoutSample
file.
Prerequisites
Before starting, you need to gather some information from the Appcharge dashboard:
- Public Publisher Token: Navigate to
Admin -> Integration -> Checkout Public Key
to get your public token.
Steps to Integrate Appcharge SDK
1. Download the SDK
Download the latest release of Appcharge's Unity SDK (contact Appcharge team). The package contains a ReadMe file including all of the needed information.
2. Import the SDK into Unity
- Select the downloaded SDK package.
- Click on
All
in the import menu, then selectImport
. This will add the Appcharge SDK code to your project.
3. Adding Appcharge's Dependencies
To add Appcharge's SDK Android dependencies to your project, you have to add a custom mainTemplate.gradle
to your project.
- Enable Custom Gradle Template
Skip this if you already have a mainTemplate.gradle
file in your project
Go to Player Settings > Android > Publishing Settings > Build > Custom Main Gradle Template
, and mark the checkbox true.
- Add Dependencies to
mainTemplate.gradle
Add the following code to your project mainTemplate.gradle
file, under dependencies
:
dependencies {
implementation fileTree(dir: 'libs', include: ['*.jar'])
implementation("androidx.browser:browser:1.6.0")
}
Please note that if Android Auto Resolver is activated, it might manipulate this file.
4. Setup the AppChargeConfig File
- Locate the
AppChargeConfig
file in the folderAssets/Resources/AppCharge
. - In case the
AppChargeConfig
file is not presented, right clickCreate > Appcharge > ConfigFile
- Configure the following settings:
Setting | Description |
---|---|
Environment | Choose your desired environment. (Either Sandbox or Production) |
Public Publisher Token | Paste your public token obtained from the Appcharge dashboard. |
Show Loader | Choose if you want to display the visual loader when loading the checkout. |
Show Success | Choose if you want to display the default success screen after a successful transaction. |
Canvas Name | Specify the name of the Canvas to be used for the Appcharge Checkout (The name of your canvas in the scene). |
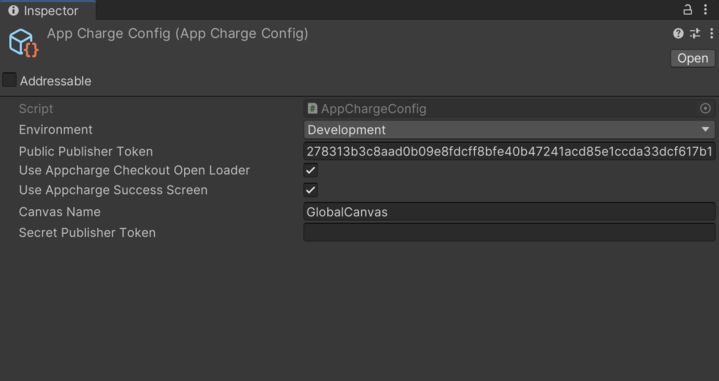
5. Initialize the SDK
Use gameObject
to initialize the SDK, preferably at the start of your game.
gameObject
can be any object in the scene. We suggest creating a new gameObject
in your scene called AppchargeManager.
Example:
// Initialize Appcharge component
AppchargeManager.Init(gameObject);
Replace gameObject
with the chosen GameObject
.
6. Listen to SDK Events
The Appcharge checkout will fire the following front-end side events to enable realtime analytics for the publisher usage. Some events are purely analytical, whereas others will require actions on the publisher side.
The table below outlines each event and the recommended actions to take when they occur (if needed):
Property name | Event name | Description | Recommended Action |
---|---|---|---|
OnLoaded | loaded | The checkout has finished loading | Close the publisher's custom loading animation (if used) |
OnOrderSuccess | orderSuccess | The publisher has acknowledged that the player's account was awarded with the purchase | Run publisher customized successful purchase animation and resume game (if used) |
OnOrderFailed | orderFail | The award has failed, although the payment was successful | Check server errors, don’t run success animation and resume game |
OnClose | close | The checkout was closed by the player | Resume game or convince the player to stay in store |
OnError | error | The checkout throws general error | Resume game or convince the player to stay in store |
To handle events from the SDK, use the following code:
AppchargeManager.ListenToEvent(CheckoutEvents.OnLoaded, (Dictionary<string, string> args) =>
{
Debug.Log("Checkout loaded triggered: " + Utils.ConvertToString(args));
});
Please refer to the Player Payment Real Time SDK Events Documentation for more information.
7. Configure Visual Loader
To use the Appcharge loader:
- Locate the
AppChargeConfig
file in the folderAssets/Resources/AppCharge
. - Turn on the loader toggle.
To use your own loader:
- Locate the
AppChargeConfig
file in the folderAssets/Resources/AppCharge
. - Turn off the loader toggle.
- Show your visual loader animation after calling
AppchargeManager.OpenCheckout
. - Upon getting the
OnLoaded
event, remove your loader.
8. Launch the Checkout
Create a checkout model by using the following code snippet:
AppChargeCheckoutModel checkoutModel = new AppChargeCheckoutModel(...);
Parameters required for the checkout model:
Parameter | Type | Description |
---|---|---|
customerId | string | The name of the customer |
customerEmail | string | Customer email address |
price | float | Offer price (in cents) |
currency | string | Offer price currency. For example: "usd", "eur" |
offerName | string | The name of the offer |
offerSku | string | Offer's sku |
offerAssetUrl | string | An image URL for the offer |
offerDescription | string | Offer's description |
Add a set of items (bundle) as followed:
Note: At least one item must be included.
// Utilize the model by adding extra items
checkoutModel.AddItem(...);
Here are the parameters needed for creating an item:
Parameter | Description |
---|---|
itemName | The name of the item |
assetUrl | An image which represents the item |
itemSku | Item's sku |
itemAmount | Item's Quantity |
Example:
// Prepare checkout model
string customerId = "JohnDoe";
string customerEmail = "[email protected]";
int price = 129; // in USD cents
string currency = "usd";
string offerName = "BestDealPackage";
string offerSku = "best_deal_package";
string offerAssetUrl = "https://media-dev.appcharge.com/media/64ad4f25cc1a482bac467ae5/fire-fire-logo-fire-fire-icon-fire-sign-fire-symbol-transparent-background-ai-generative-free-png.png";
string offerDescription = "Coin Pack Bundle";
// Declare a model for getting session token
checkoutModel = new RequestModel(
customerId,
customerEmail,
price,
currency,
offerName,
offerSku,
offerAssetUrl,
offerDescription
);
// Utilize the model by adding extra items
string itemName = "Coins";
string itemAssetUrl = "https://media-dev.appcharge.com/media/product-3.png";
string itemSku = "coins";
int itemAmount = 300;
checkoutModel.AddItem(itemName, itemAssetUrl, itemSku, itemAmount);
Open the checkout using the checkoutModel
:
AppchargeManager.OpenCheckoutDirect(checkoutModel);
This function should be called when a user presses a button in your in-game store and once you initialized the checkoutModel
.
9. Configure Success Screen and Close Checkout
To use the Appcharge success screen:
- Locate the
AppChargeConfig
file in the folderAssets/Resources/AppCharge
. - Turn on the success screen toggle.
- Listen to the
CheckoutEvents.OnOrderSuccess
event, and wait 1.5 seconds for the animation to finish running. - Close the Appcharge SDK using the
AppchargeManager.Close
function.
To use your own Success screen:
- Locate the
AppChargeConfig
file in the folderAssets/Resources/AppCharge
. - Turn off the success screen toggle.
- Listen to the
CheckoutEvents.OnOrderSuccess
event. - Close the Appcharge SDK using the
AppchargeManager.Close
function. - Show your own success screen. For example:
AppchargeManager.ListenToEvent(CheckoutEvents.OnOrderSuccess, (Dictionary<string, string> args) =>
{
Debug.Log("Checkout Success triggered");
AppchargeManager.Close(); // Close the checkout
});